If you've been using Git and running git add and git commit frequently, you might wonder:
"How can I check all the versions I've created?"
The answer is simple: Use git log!
1️⃣ What is git log?
The git log command allows you to see all the commit history in your repository.
Simply type the following command:
git log
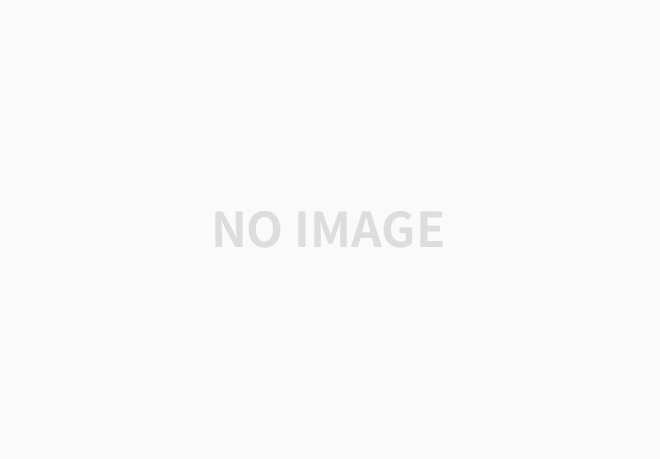
This will display details about each commit, including:
✅ Commit hash → A unique ID for each commit
✅ HEAD info → Shows which branch you’re currently on
✅ Author information → Useful for collaboration
✅ Commit message → A short description of the changes
📌 Why is the commit hash important?
Each commit has a long hash (e.g., a1b2c3d4...).This is useful when you need to switch to a specific version later.
2️⃣ Making git log More Readable
By default, git log displays a lot of information, which can be overwhelming.
Here are two useful options to make it more readable.
🔹 1. --graph (Visualizing commit relationships)
git log --graph
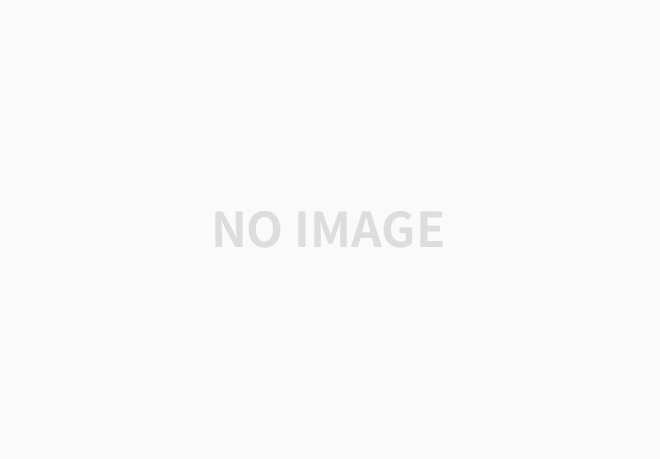
This option draws a graph to show how commits are connected.
It becomes extremely useful when working with branches.
🔹 2. --oneline (Displaying commits in a single line)
git log --oneline
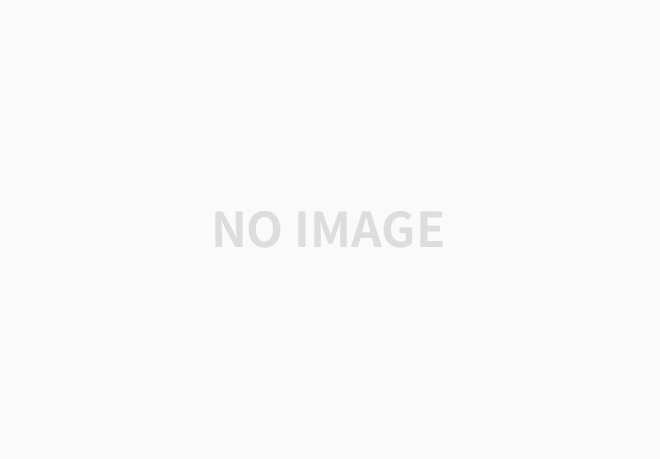
This command simplifies the output by showing only the commit hash (7 characters) and message.
🔹 3. --graph + --oneline (Best combination)
git log --graph --oneline
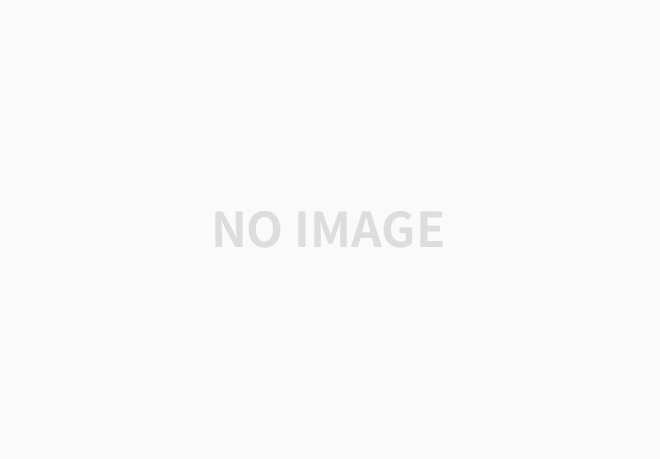
This is the most commonly used combination to keep logs simple and structured.
🎯 Summary
✔ git log helps you review your commit history.
✔ Use --graph and --oneline to make it easier to read.
Git logs are essential when you need to track changes, revert to previous versions, or collaborate with a team.
'프로그래밍 > GIT' 카테고리의 다른 글
How to Use Git Reset & Restore for Version Management (Undoing Previous Commits) (0) | 2025.03.30 |
---|---|
Git Rebase & Squash로 깔끔하게 브랜치 정리하는 방법 (0) | 2025.03.29 |
(1) Git Add, Commit, and Version Control (Creating Repositories and Versions) (0) | 2025.03.24 |
What is Git? (0) | 2025.03.23 |
(3-4) git merge란?(어떤 branch가 남고 어떤 branch가 없어지지?) (0) | 2024.05.16 |